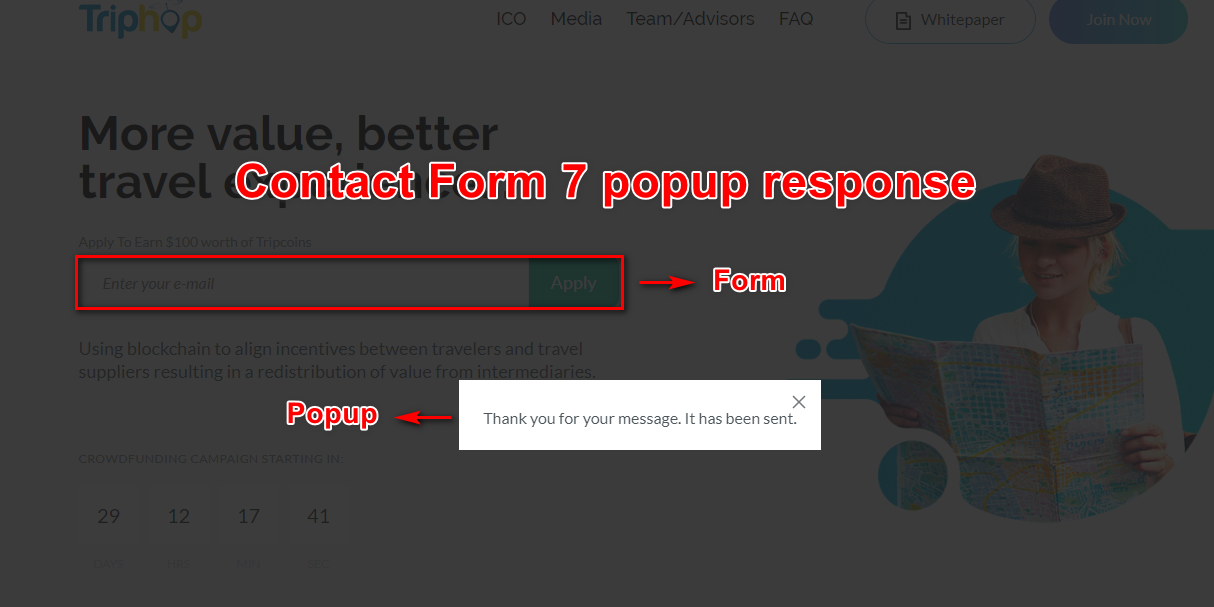
Why we are not using any plugin to show the Contact Form 7 popup success message? Because it is really simple to do it without plugins and in the result we can have one popup for all forms or separate popups for all forms, modals can have any design you like and so on. Usually, plugins allow you to configure options than they have and it is almost impossible to configure what you need.
In our case, we will display one popup for all Contact form 7 successful messages.
For displaying Contact Form 7 messages with a popup we are going to use Fancybox 3. You can integrate Fancybox in your custom theme (child theme) or plugin separately from other styles and scripts, but we are using SASS and compile all styles and scripts into two files with Gulp.js: app.css and app.js. This is the code to implement them to your custom theme:
/**
* Enqueue scripts and styles.
*/
function wp_enqueue_scripts_func() {
if ( !is_admin() ) {
wp_enqueue_style( 'theme-css', get_template_directory_uri() . '/dist/css/app.css', array(), '' );
// Deregister the jquery version bundled with WordPress.
wp_deregister_script( 'jquery' );
// CDN hosted jQuery placed in the header, as some plugins require that jQuery is loaded in the header.
wp_enqueue_script( 'jquery', get_template_directory_uri() . '/dist/js/jquery.min.js', array(), '3.3.1', false );
// Deregister the jquery-migrate version bundled with WordPress.
wp_deregister_script( 'jquery-migrate' );
// CDN hosted jQuery migrate for compatibility with jQuery 3.x
wp_register_script( 'jquery-migrate', '//code.jquery.com/jquery-migrate-3.0.1.min.js', array('jquery'), '3.0.1', false );
wp_enqueue_script( 'theme-script', get_template_directory_uri() . '/dist/js/app.js', array('jquery'), '', true );
wp_localize_script( 'theme-script', 'theme', array(
'url' => home_url(),
'themeUrl' => get_template_directory_uri(),
'ajaxurl' => admin_url( 'admin-ajax.php' )
) );
}
}
add_action( 'wp_enqueue_scripts', 'wp_enqueue_scripts_func' );
It is pretty easy to install Contact Form 7 plugin into your WordPress website and to configure form you’d like to show to your visitors. We’ll skip these steps. Now after filling the form and submitting it people can get success or error messages. We will show the success message inside a lightbox and we’ll not display a modal for error messages. It is better to process them with regexp to make them look good and not repeated. We can do this but the idea of this article appears when we were developing a website for one of our customers and it was needed. So to display that fields of a form are not correct we will not display any messages and we’ll just make a red border for wrong inputs. It’s enough if your form consists of for example two fields (name and email).
/**
* Display Contact Form 7 messages in a popup.
*/
function ContactForm7_popup() {
$return = <<<EOT
<script>
jQuery(".wpcf7-form input[type='submit'], .wpcf7-form button").click(function(event) {
jQuery( document ).one( "ajaxComplete", function(event, xhr, settings) {
var data = xhr.responseText;
var jsonResponse = JSON.parse(data);
// console.log(jsonResponse);
if(! jsonResponse.hasOwnProperty('into') || $('.wpcf7' + jsonResponse.into).length === 0) return;
// alert(jsonResponse.message);
$.fancybox.open(
'<div class="message">' + jsonResponse.message + '</div>',
{
smallBtn : true,
toolbar : false
}
);
});
});
</script>
<style>
div.wpcf7-response-output, div.wpcf7-validation-errors { display: none !important; }
span.wpcf7-not-valid-tip { display: none; }
input[aria-invalid="true"], select[aria-invalid="true"] { border-color: #ff2c00; // background-color: rgba(153,0,0,0.3); }
</style>
EOT;
echo $return;
}
add_action( 'wp_footer', 'ContactForm7_popup', 20 );
Easy, isn’t it? As I said before adding some conditions you can use this code to show Contact Form 7 popup response for form with exact ID or to show error messages inside a popup, too, also you can choose any design, timeout to make this popup disappear.
Hope my ideas were helpful and inspiring for you. Bye to all!
https://wordpress.org/plugins/popup-message-for-contact-form-7/ you can use this script that just simple
Yes, but there is not so powerful as the decision we described here. At the beginning of the article we explained why we don’t like to use a plugin to display response from contact form 7 in a popup.
https://wordpress.org/plugins/popup-message-contact-form-7/ This is also good for failed and success both for setup popup with mobile friendly