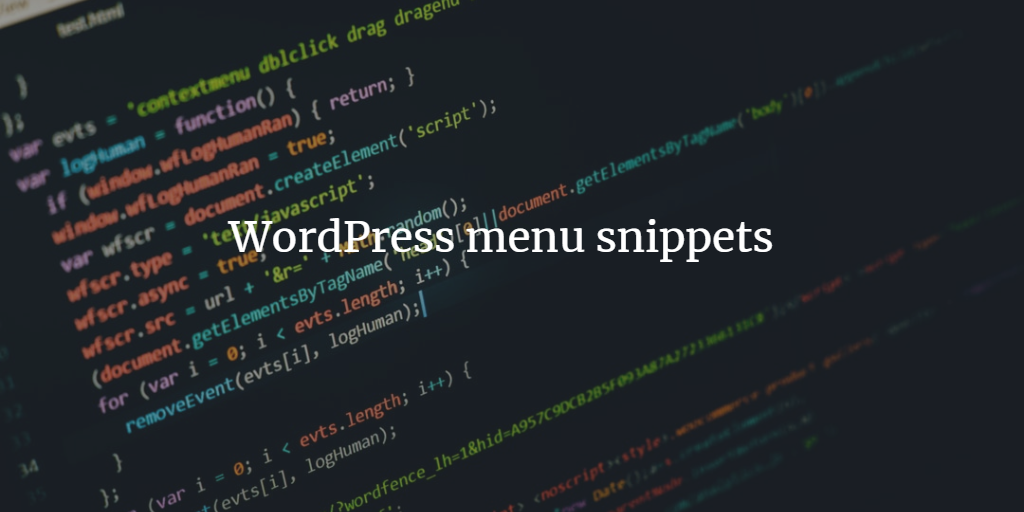
The development of custom menu may sound a little frightening, but often using plugins for the menu will increase the number of plugins, decrease page loading speed and doesn’t allow to create the menu that will fit the design. Here you are some WordPress menu snippets that we like to use.
Displaying the menu in WordPress custom themes.
The most common way to display a menu in your theme is to register theme location for your menu. You should put this code inside functions.php.
/**
* Register for theme menu.
*/
function theme_register_menu() {
register_nav_menu( 'primary', 'Primary Menu' );
register_nav_menu( 'settings', 'Settings Menu' );
}
add_action( 'after_setup_theme', 'theme_register_menu', 11 );
Then you can show menu within theme template (usually header.php) with code:
/**
* Displaying the theme menu.
*/
$args = array(
'menu' => 'primary',
'menu_id' => 'main_menu',
'theme_location' => 'primary',
'container' => ''
);
wp_nav_menu( $args );
To remove a link from a menu and to display menu item as text you can use this:
All snippets below you will need to put in the functions.php file.
/**
* Adding span to a menu.
* Class text allows wrapping menu item within span tag instead of a tag.
* Class disable allows to remove menu item wrapper at all.
*/
function span_to_nav_menu( $item_output, $item, $depth, $args ) {
if ( isset( $item->classes ) && !empty( $item->classes ) ) {
if ( in_array( 'text', (array) $item->classes ) ) {
$item_output = '<span class="title">'. $item->title .'</span>';
} elseif ( in_array( 'disable', (array) $item->classes ) ) {
$item_output = '';
}
}
return $item_output;
}
add_filter( 'walker_nav_menu_start_el', 'span_to_nav_menu', 10, 4 );
You can insert a shortcode it menu item title with code:
/**
* Allow shortcodes in a menu in the title.
*/
add_filter('wp_nav_menu_items', 'do_shortcode');
To add HTML code or shortcodes to a menu in WordPress use this snippet:
/**
* Allow HTML and shortcodes in a menu in the description.
*/
remove_filter( 'nav_menu_description', 'strip_tags' );
function my_plugin_wp_setup_nav_menu_item( $menu_item ) {
if ( ! is_admin() && isset( $menu_item->post_type ) ) {
if ( 'nav_menu_item' == $menu_item->post_type ) {
$menu_item->description = do_shortcode($menu_item->post_content);
}
}
return $menu_item;
}
add_filter( 'wp_setup_nav_menu_item', 'my_plugin_wp_setup_nav_menu_item' );
This code will help you to wrap submenus of the menu.
Do not forget to specify walker in arguments when you are displaying menu ‘walker’ => new Custom_Sublevel_Walker
/**
* Extending menu walker. Allow to wrap submenus. Just an example.
*/
class Custom_Sublevel_Walker extends Walker_Nav_Menu
{
function start_lvl( &$output, $depth = 0, $args = array() ) {
$indent = str_repeat("\t", $depth);
if ( $depth == 0 ) {
$output .= "\n$indent<div class='sub-menu-wrap first_level_menu'><ul class='sub-menu row'>\n";
} elseif( $depth == 1 ) {
$output .= "\n$indent<div class='sub-menu-wrap second_level_menu'><ul class='sub-menu row'>\n";
} elseif( $depth == 2 ) {
$output .= "\n$indent<div class='sub-menu-wrap third_level_menu'><ul class='sub-menu'>\n";
} else {
$output .= "\n$indent<div class='sub-menu-wrap fourth_level_menu'><ul class='sub-menu'>\n";
}
}
function end_lvl( &$output, $depth = 0, $args = array() ) {
$indent = str_repeat("\t", $depth);
$output .= "$indent</ul></div>\n";
}
}
Hope you will find menu snippets in this article useful and will give us a good feedback) If not say us about your issue and we will try to help you.